How To Change Character In String In Python
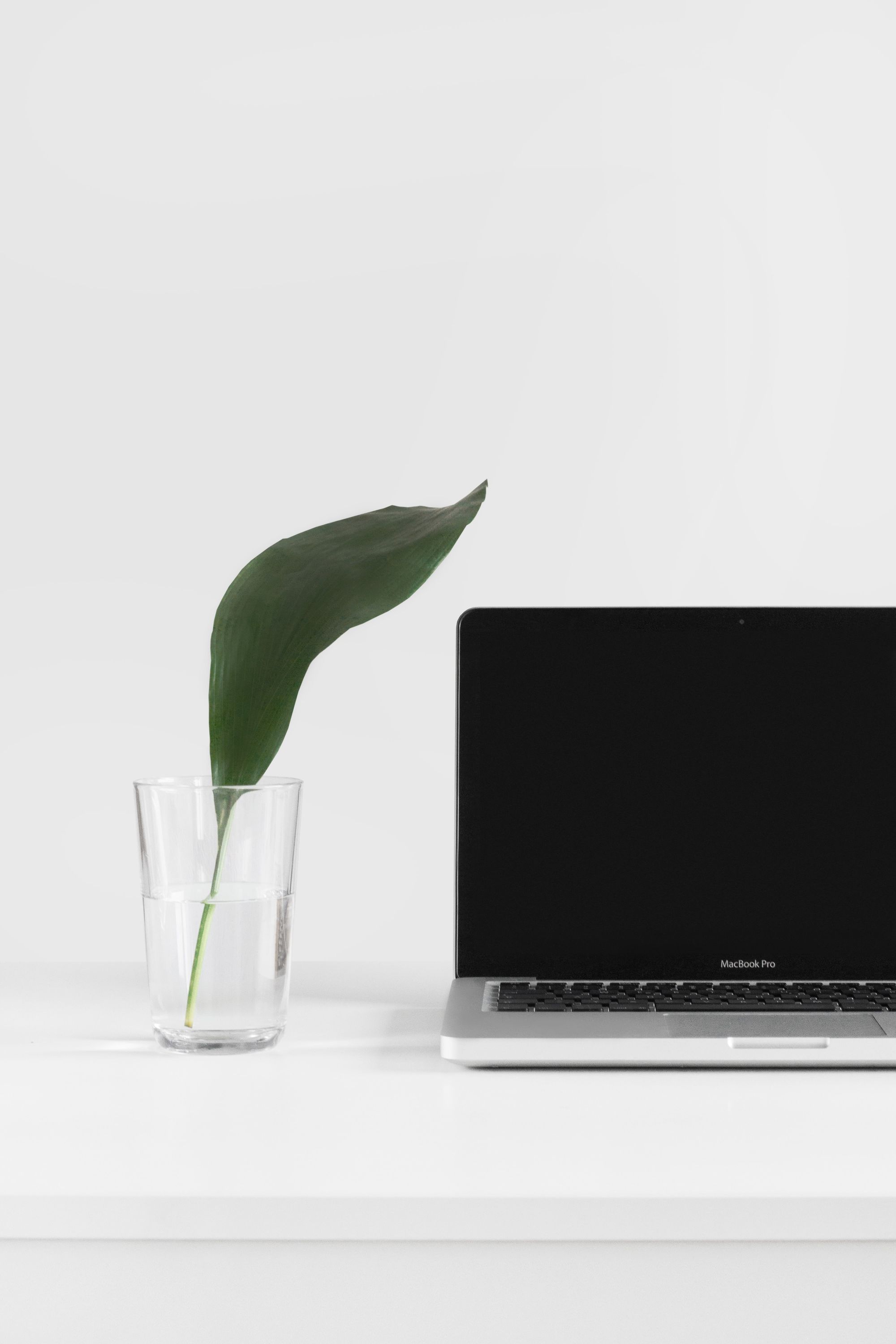
In this article you'll run across how to use Python's .replace()
method to perform substring substiution.
You'll also meet how to perform instance-insensitive substring substitution.
Let'due south get started!
What does the .replace()
Python method do?
When using the .supplant()
Python method, yous are able to replace every instance of one specific character with a new i. You lot can fifty-fifty supplant a whole cord of text with a new line of text that you specify.
The .replace()
method returns a copy of a string. This means that the old substring remains the same, but a new copy gets created – with all of the quondam text having been replaced by the new text.
How does the .replace()
Python method work? A Syntax Breakdown
The syntax for the .supplant()
method looks like this:
cord.supervene upon(old_text, new_text, count)
Let'south break it downwards:
-
old_text
is the first required parameter that.replace()
accepts. Information technology'southward the sometime graphic symbol or text that you lot want to replace. Enclose this in quotation marks. -
new_text
is the 2nd required parameter that.replace()
accepts. It's the new graphic symbol or text which you desire to supervene upon the old character/text with. This parameter also needs to exist enclosed in quotation marks. -
count
is the optional third parameter that.supercede()
accepts. Past default,.supervene upon()
will supercede all instances of the substring. Still, yous can usecount
to specify the number of occurrences you want to exist replaced.
Python .supersede()
Method Code Examples
How to Supervene upon All Instances of a Unmarried Grapheme
To change all instances of a single grapheme, yous would do the post-obit:
phrase = "I like to learn coding on the go" # replace all instances of 'o' with 'a' substituted_phrase = phrase.supplant("o", "a" ) print(phrase) print(substituted_phrase) #output #I like to larn coding on the go #I similar ta learn cading an the ga
In the instance above, each word that independent the graphic symbol o
is replaced with the graphic symbol a
.
In that example in that location were iv instances of the character o
. Specifically, information technology was found in the words to
, coding
, on
, and get
.
What if y'all but wanted to alter two words, like to
and coding
, to contain a
instead of o
?
How to Replace Only a Certain Number of Instances of a Unmarried Character
To change simply two instances of a single character, y'all would use the count
parameter and set it to two:
phrase = "I like to learn coding on the get" # replace merely the first 2 instances of 'o' with 'a' substituted_phrase = phrase.replace("o", "a", 2 ) print(phrase) print(substituted_phrase) #output #I like to acquire coding on the go #I like ta learn cading on the go
If you only wanted to change the offset example of a single character, you would gear up the count
parameter to one:
phrase = "I like to learn coding on the get" # replace just the first instance of 'o' with 'a' substituted_phrase = phrase.supersede("o", "a", ane ) print(phrase) print(substituted_phrase) #output #I like to learn coding on the become #I like ta learn coding on the go
How to Supervene upon All Instances of a String
To change more than one character, the process looks similar.
phrase = "The lord's day is strong today. I don't really like sun." #supersede all instances of the word 'sun' with 'air current' substituted_phrase = phrase.replace("sunday", "current of air") print(phrase) impress(substituted_phrase) #output #The lord's day is strong today. I don't really like sun. #The wind is strong today. I don't really similar wind.
In the example higher up, the word sun
was replaced with the word wind
.
How to Replace Simply a Certain Number of Instances of a String
If you wanted to change just the get-go instance of sun
to wind
, yous would use the count
parameter and ready it to i.
phrase = "The sun is strong today. I don't really like sun." #replace but the first instance of the discussion 'dominicus' with 'wind' substituted_phrase = phrase.supercede("dominicus", "air current", 1) print(phrase) print(substituted_phrase) #output #The sun is stiff today. I don't really like sun. #The wind is strong today. I don't actually like dominicus.
How to Perform Example-Insensitive Substring Substitution in Python
Allow's have a look at another instance.
phrase = "I am learning Ruby. I actually enjoy the cherry-red programming language!" #replace the text "Ruby" with "Python" substituted_text = phrase.supercede("Ruby", "Python") impress(substituted_text) #output #I am learning Python. I really bask the ruby programming linguistic communication!
In this example, what I actually wanted to do was to replace all instances of the word Ruby-red
with Python
.
However, there was the word ruby-red
with a lowercase r
, which I would also similar to alter.
Considering the start letter was in lowercase, and not uppercase as I specified with Cherry-red
, it remained the same and didn't change to Python
.
The .replace()
method is case-sensitive, and therefore it performs a case-sensitive substring substitution.
In order to perform a case-insensitive substring substitution you would have to practice something different.
You lot would need to use the re.sub()
function and use the re.IGNORECASE
flag.
To use re.sub()
you need to:
- Utilize the
re
module, viaimport re
. - Speficy a regular expression
blueprint
. - Mention with what you lot want to
replace
the pattern. - Mention the
string
you want to perform this performance on. - Optionally, specify the
count
parameter to make the replacement more precise and specify the maximum number of replacements you want to accept place. - The
re.IGNORECASE
flag tells the regular expression to perform a case-insensitive match.
So, all together the syntax looks like this:
import re re.sub(pattern, replace, string, count, flags)
Taking the instance from earlier:
phrase = "I am learning Ruby. I actually relish the ruddy programming language!"
This is how I would replace both Ruby
and ruddy
with Python
:
import re phrase = "I am learning Ruby. I really enjoy the ruby programming linguistic communication!" phrase = re.sub("Cherry","Python", phrase, flags=re.IGNORECASE) impress(phrase) #output #I am learning Python. I really savor the Python programming language!
Wrapping upwardly
And there you have it - you at present know the basics of substring substitution. Hopefully you found this guide helpful.
To learn more about Python, check out freeCodeCamp'due south Scientific Computing with Python Certification.
You lot'll beginning from the basics and larn in an interacitve and beginner-friendly way. You'll also build 5 projects at the end to put into practice and help reinforce what you learned.
Thanks for reading and happy coding!
Learn to lawmaking for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people get jobs as developers. Get started
Source: https://www.freecodecamp.org/news/python-string-replace-function-in-python-for-substring-substitution/
Posted by: pilgrimanable.blogspot.com
0 Response to "How To Change Character In String In Python"
Post a Comment